Error Call to a Member Function getCollectionParentid() on Null:
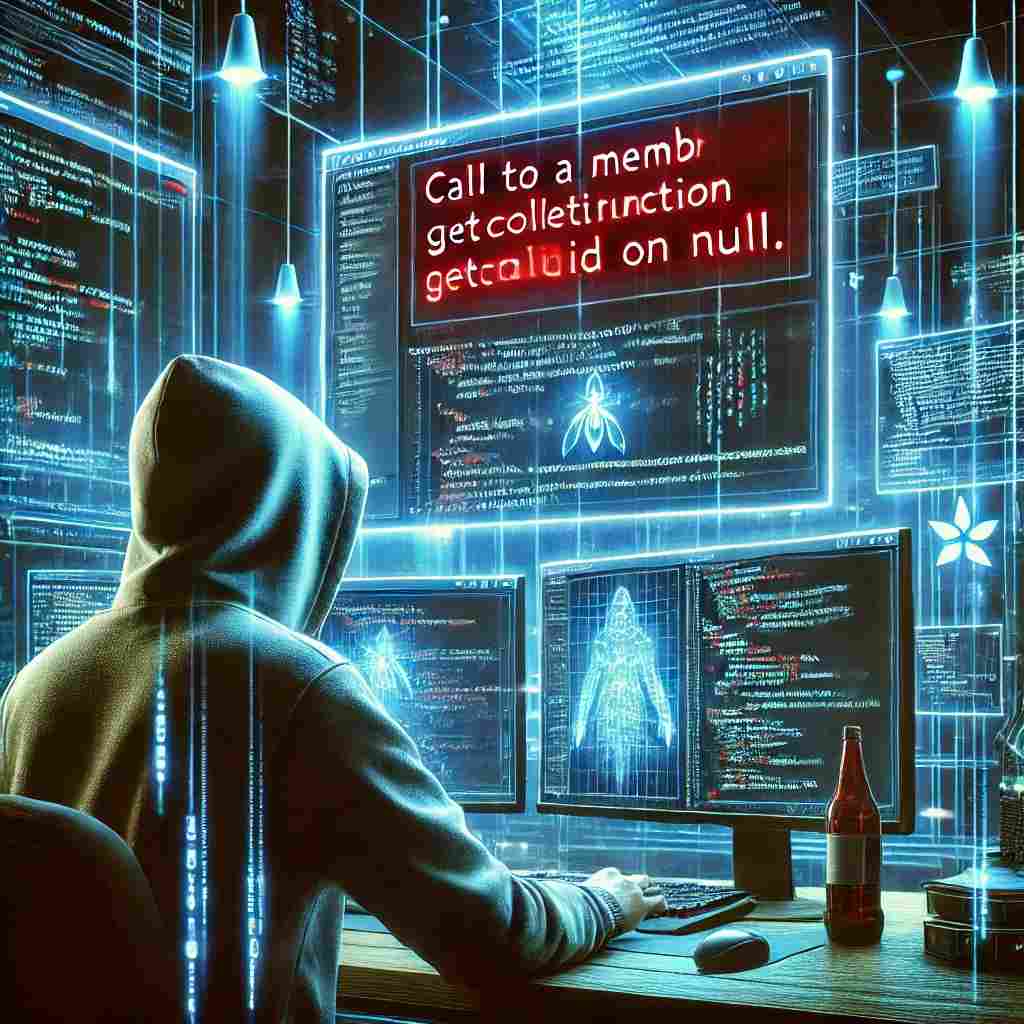
Introduction:
The error “Call to a Member Function getCollectionParentId() on Null” is a common PHP runtime issue that arises when your code tries to invoke a method on an uninitialized object. This problem can interrupt your application, degrade user experience, and lead to delays in debugging.
In this article, we’ll explore the causes, diagnosis methods, and practical solutions to address this error.
What is the Error: Call to a Member Function getCollectionParentId() on Null?
This error means your code is trying to call the getCollectionParentId() method on an object that hasn’t been properly instantiated or initialized.
Essentially, the object being referenced is null, which means it doesn’t exist or hasn’t been assigned a valid value.
Common Causes of the Error: Call to a Member Function getCollectionParentId() on Null
- Uninitialized Objects:
An object must be properly initialized before you can call methods on it. If the object is null, attempting to use its methods will trigger this error.
- Database Connection Issues:
If your application relies on database queries to initialize objects, failure to connect to or retrieve data from the database can result in null values.
- Incorrect Method Calls:
Calling methods that don’t exist or using incorrect names can lead to this error when the expected object isn’t correctly assigned.
- Missing Dependencies:
Dependencies not loaded properly can result in objects being null, as the necessary components aren’t available.
- Incorrect Object Hierarchies:
Misconfigured object relationships or improper hierarchy definitions can cause your code to reference null objects.
Diagnosing the Error: Call to a Member Function getCollectionParentId() on Null
Step 1: Identify the Error Location
Check the error message and stack trace to pinpoint the file and line where the error occurs. This is crucial for understanding the context of the problem.
Step 2: Check Object Initialization
Inspect the code to verify that the object calling getCollectionParentId() is properly initialized and not null.
Step 3: Review Database Queries
If the object depends on data from the database, review your queries to ensure they are returning valid results.
Step 4: Validate Method Calls
Ensure the getCollectionParentId() method exists in the class and is invoked correctly.
Step 5: Inspect Dependencies
Make sure all necessary dependencies are properly included and loaded in the application.
Step 6: Analyze Object Hierarchies
Verify the structure and relationships of objects to confirm they are configured correctly.
Solutions to Fix the Error: Call to a Member Function getCollectionParentId() on Null
Solution 1: Proper Object Initialization
Always initialize objects before using their methods:
php
Copy code
if ($object !== null) {
$parentId = $object->getCollectionParentId();
} else {
// Handle the null case appropriately
}
Solution 2: Database Connection Verification
Ensure your application can connect to the database and retrieve data as expected. Test the queries to verify they return the desired results.
Solution 3: Correct Method Calls
Double-check that the getCollectionParentId() method exists in the class and that the correct syntax is used:
php
Copy code
if (method_exists($object, ‘getCollectionParentId’)) {
$parentId = $object->getCollectionParentId();
} else {
// Handle the missing method scenario
}
Solution 4: Load Dependencies Properly
Ensure all required dependencies and classes are loaded. Use autoloaders or manually include necessary files:
php
Copy code
require_once ‘path/to/dependency.php’;
Solution 5: Manage Object Hierarchies
Verify and manage object hierarchies to ensure correct relationships and avoid null references.
Preventive Measures to Avoid the Error: Call to a Member Function getCollectionParentId() on Null
- Implement Error Handling:
Use try-catch blocks to gracefully handle exceptions and null cases:
php
Copy code
try {
$parentId = $object->getCollectionParentId();
} catch (Exception $e) {
// Log error and provide fallback
}
- Validate Data Retrieval:
Always validate the data retrieved from the database or external sources before using it.
- Use Dependency Injection:
Leverage dependency injection to manage dependencies effectively and ensure proper initialization.
- Follow Best Practices
Adopt coding best practices such as adhering to naming conventions, validating inputs, and structuring code logically.
- Regular Code Reviews:
Conduct periodic code reviews to identify and address potential issues before they escalate.
FAQ’s
1. What causes the “Call to a Member Function getCollectionParentId() on Null” error?
This error is typically caused by attempting to call a method on an uninitialized or null object, often due to database issues, missing dependencies, or incorrect method calls.
2. How can I diagnose the “Call to a Member Function getCollectionParentId() on Null” error?
You can diagnose the error by examining the stack trace, checking object initialization, reviewing database queries, and ensuring method validity.
3. What are some solutions to fix the “Call to a Member Function getCollectionParentId() on Null” error?
Solutions include initializing objects properly, validating database queries, ensuring correct method usage, loading dependencies, and managing object hierarchies.
4. How can I prevent the “Call to a Member Function getCollectionParentId() on Null” error?
Prevent this error by implementing robust error handling, validating inputs, conducting code reviews, and using dependency injection.
5. Why is error handling vital in preventing the “Call to a Member Function getCollectionParentId() on Null” error?
Error handling helps manage unexpected situations gracefully, preventing application crashes and ensuring a seamless user experience.
Conclusion:
The “Call to a Member Function getCollectionParentId() on Null” error is a common issue that disrupts applications but is entirely preventable. By identifying its causes, systematically diagnosing problems, and applying effective solutions like proper object initialization and database checks, you can resolve it efficiently.
Implementing preventive measures such as robust error handling, dependency injection, and regular code reviews ensures greater application reliability and a seamless user experience.